
|
FIFO
/*Fist In First Out Page Replacement Algoritham*/
/*~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~*/
#include<stdio.h>
#include<conio.h>
#include<stdlib.h>
int f[3],ptr=0,page_fault=0;
void main()
{
int temp,st;
char ch;
void display();
int is_memoryfull();
int checkitem(int);
clrscr();
while(1)
{
printf("enter the page number\n");
scanf("%d",&temp);
if(checkitem(temp))
{
st=is_memoryfull();
if(st==1)
++page_fault;
f[ptr]=temp;
++ptr;
if(ptr==3)
ptr=0;
}
display();
printf("do u want to continue (y/n) :");
scanf(" %c", &ch);
if(ch=='n')
exit(1);
}
}
void display()
{
printf("pages in memory\n");
for(int i=0;i<3;++i)
printf("%d\n",f[i]);
printf("no of page faults is: %d\n",page_fault);
}
int checkitem(int t)
{
for(int j=0;j<3;++j)
if(t==f[j])
return(0);
return(1);
}
int is_memoryfull()
{
for(int k=0;k<3;++k)
if(f[k]==0)
return(0);
return(1);
}
|

|
SJF
/* Shotest Job First Algoritham */
/*~~~~~~~~~~~~~~~~~~~~~~~~~~~~~*/
#include<stdio.h>
#include<conio.h>
void main()
{
struct sfj
{
int id,wt,tat,st;
}f[20];
int i,j,n,temp;
clrscr();
printf("enter the no of jobs");
scanf("%d",&n);
printf("enter the id's of all jobs\n");
for(i=0;i<n;++i)
scanf("%d",&f[i].id);
printf("enter service time of all jobs\n");
for(i=0;i<n;++i)
scanf("%d",&f[i].st);
/* The bellow code used to sort the srvice times of jobs and theire id's */
for(j=0;j<n;++j)
{
for(i=0;i<n-1;++i)
{
if(f[i].st>f[i+1].st)
{
temp=f[i].st;
f[i].st=f[i+1].st;
f[i+1].st=temp;
temp=f[i].id;
f[i].id=f[i+1].id;
f[i+1].id=temp;
}
}
}
for(i=0;i<n;++i)
printf("%d %d\n",f[i].id,f[i].st);
printf("\n\n");
f[0].wt=0;
f[0].tat=f[0].st;
for(i=1;i<n;i++)
{
f[i].wt=f[i-1].tat;
f[i].tat=f[i].wt+f[i].st;
}
printf("id \t st \t wt \t tat \n");
for(i=0;i<n;++i)
{
printf("%d\t",f[i].id);
printf("%d\t",f[i].st);
printf("%d\t",f[i].wt);
printf("%d \t",f[i].tat);
printf("\n");
}
getch();
}
|
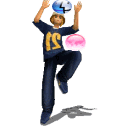
|
Optimal Page Replacement Technic
/* Optimal Page Replacement Technic */
/*~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~*/
#include<stdio.h>
#include<conio.h>
int i=0,k;
int a[13]={1,4,3,6,2,8,3,4,6,1,3,8,4}; /* a[] is the array it holds the */
/* set of page numbers. */
int s[3]; /* s[] is the array it contains frames in which */
void main() /* pages can be Replaced. */
{
int j=0,longest,t;
int check(int k);
int f(int ,int);
void display();
clrscr();
while(i!=3)
{
s[j]=a[i];
++i,++j;
}
printf("page no in frames\n");
display();
for(int x=3;x<13;++x)
{
t=a[i];
++i;
if(check(t))
{
if(f(s[0],i)==0)
s[0]=t;
else
if(f(s[1],i)==0)
s[1]=t;
else
if(f(s[2],i)==0)
s[2]=t;
else
{
if(f(s[0],i) > f(s[1],i))
longest=f(s[0],i);
else
if(f(s[1],i) >f(s[2],i))
longest=f(s[1],i);
else
longest=f(s[2],i);
for(int k=0;k<3;++k)
if(a[longest]==s[k])
s[k]=t;
}
}
display();
}
getch();
}
int check(int t)
{
for(int i=0;i<3;++i)
if(t==s[i])
return(0);
return(1);
}
void display()
{
for(int i=0;i<3;++i)
printf(" %d\n",s[i]);
printf("\n");
}
int f(int j,int i)
{
for(int k=i;k<13;++k)
if(a[k]==j)
return(k);
return(0);
}
|
|
Round Robin
/* Round Robin fsPage Replacement Algoritham */
/*~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~*/
#include<stdio.h>
#include<conio.h>
int count=0,n,k=0;
void main()
{
int st[20],f[20];
int q=2;
int i;
int checkstr(int a[]);
clrscr();
printf("enter the no processes\n");
scanf("%d",&n);
printf("\nEnter the servise of time of %d processe:\n",n);
for(i=0;i<n;++i)
scanf("%d",&st[i]);
while(checkstr(st))
{
for(i=0;i<n;++i)
if(st[i]>q)
{
st[i]=st[i]-q;
count=count+q;
}
else
{
if(st[i]!=0)
{
count=count+st[i];
st[i]=k;
f[i]=count;
}
}
}
printf("finish times");
for(i=0;i<n;++i)
printf("ft[%d] is %d \n",i,f[i]);
getch();
}
int checkstr(int st[])
{
int i=0;
for(i=0;i<n;++i)
if(st[i]!=0)
return(1);
return(0);
}
|
|
BANKERS ALGORITHM
/*BANKERS ALGORITHM FOR AVOIDANCE OF DEADLOCK*/
/*~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~*/
#include<stdio.h>
#include<conio.h>
#include<process.h>
int rsc[3]={9,3,6};
int avail[3]={3,3,3};
int alloc[4][3]={1,0,0,6,1,2,2,1,1,0,0,2};
int claim[4][3]={3,2,2,6,1,3,3,1,4,4,2,2};
int req[3];
int x,i,r;
void main()
{
int k,j;
void display();
int safe();
clrscr();
display();
printf("which process is requesting now \n");
scanf("%d",&r);
for(x=0;x<3;++x)
scanf("%d",&req[x]);
for(i=0;i<3;++i)
if(alloc[r-1][i]+req[i] > claim[r-1][i])
{
printf("error is: it is requesting more than required resources \n");
exit(1);
}
for(i=0;i<3;++i)
if(req[i]>avail[i])
{
printf("it is requesting more than available resources \n");
exit(1);
}
for(i=0;i<3;++i)
{
alloc[r-1][i]=alloc[r-1][i]+req[i];
avail[i]=avail[i]-req[i];
}
printf("before entering into safe() \n");
display();
printf("after entering into safe() \n");
if(safe())
{
printf("\nNow it is in safe state, you can carry on this allocation\n");
}
else
printf("\n it is not in the safe state ,please suspend the process \n");
display();
getch();
}
void display()
{
int i,j1,j,j2,j3;
printf("claim allocation available resource \n \n");
for(i=0;i<4;++i)
{
for(j=0;j<3;++j)
printf(" %d ",claim[i][j]);
printf(" ");
for(j1=0;j1<3;++j1)
printf("%d ",alloc[i][j1]);
if(i==0)
{
printf(" ");
for(j2=0;j2<3;++j2)
printf("%d ",avail[j2]);
printf(" ");
for(j3=0;j3<3;++j3)
printf("%d ",rsc[j3]);
}
printf("\n");
}
}
int safe()
{
//int temp[4]={1,1,1,1};
int f=0,k;
int rsc1[3];
int avail1[3];
int alloc1[4][3];
int claim1[4][3];
//int flag=1;
int i,j;
for(j=0;j<3;++j)
{
rsc1[j]=rsc[j];
avail1[j]=avail[j];
}
for(i=0;i<4;++i)
for(j=0;j<3;++j)
{
alloc1[i][j]=alloc[i][j];
claim1[i][j]=claim[i][j];
}
for(i=0;i<4;++i)
{
f=0;
for(j=0;j<3;++j)
if(claim1[i][j]-alloc1[i][j]<=avail1[j])
f=f+j;
if(f==3)
return(1);
}
return(0);
}
|
|
FCFS
/* First Come First Serve Process Scheduling */
/*~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~*/
#include<stdio.h>
#include<conio.h>
void main()
{
char p[6]={'A','B','C','D','E'};
int at[10]={0,2,4,6,8};
int st[10]={3,6,4,5,2};
int ft[10],tat[10],i;
clrscr();
ft[0]=st[0];
printf("process name :");
for(i=0;i<5;i++)
printf(" %c",p[i]);
printf("\n\n");
for(i=1;i<5;i++)
ft[i]=st[i]+ft[i-1];
printf("Finishing time :");
for(i=0;i<5;i++)
printf(" %d",ft[i]);
printf("\n\n");
printf("Turnaround Time :");
for(i=0;i<5;i++)
{
tat[i]=ft[i]-at[i];
printf(" %d",tat[i]);
}
getch();
}
|

|
LRU PAGE REPLACEMENT
/*LRU PAGE REPLACEMENT ALGORITHM */
/*~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~*/
#include<stdio.h>
#include<conio.h>
#include<stdlib.h>
int a[3],i=0,st[3];
void main()
{
char ch;
int n,k,z,x,temp;
int status();
int check(int t);
void display();
clrscr();
printf("enter the elements \n");
for(k=0;k<3;++k)
{
scanf("%d",&a[k]);
st[k]=i;
++i;
}
while(1)
{
printf("\n enter the element :");
scanf("%d",&temp);
x=check(temp);
if(x!=4)
st[x]=i;
else
{
z=status();
a[z]=temp;
st[z]=i;
}
display();
++i;
printf("\n do u want to continue (y/n) :");
scanf(" %c", &ch);
if(ch=='n')
exit(1);
}
}
int status()
{
if(st[0]<st[1])
if(st[0]<st[2])
return(0);
else
return(2);
else
if(st[1]<st[2])
return(1);
else
return(2);
}
void display()
{
int i;
for(i=0;i<3;++i)
{
printf("\n");
printf("%d",a[i]);
}
}
int check(int t)
{
int i;
for(i=0;i<3;++i)
if(a[i]==t)
return(i);
return(4);
}
|

|
PAGING
#include<stdio.h>
#include<conio.h>
#include<stdlib.h>
int mem[50];
int z=0,count=0;
struct paging
{
char proc;
int pt;
int n;
};
struct page_t
{
int p[20];
int f[20];
};
paging pg[5];
page_t pgt[5];
void main()
{
int choice;
void display();
void delproc();
void pageload();
clrscr();
while(1)
{
printf("\n Enter the choice");
printf("\n Loading a new process press 1 ");
printf("\n Deleting process from memory press 2 ");
printf("\n Display the memory and pagetable press 3");
printf("\n Do u want to terminate this programm press 4\n");
scanf("%d",&choice);
switch(choice)
{
case 1:pageload();
break;
case 2:delproc();
break;
case 3:display();
break;
case 4:exit(1);
}
}
}
void display()
{
int n,pt1;
printf("page no in memory ");
for(int j=0;j<20;++j)
printf("%d ",mem[j]);
for(int i=0;i<z;++i)
{
printf("\npage table of process : ");
printf("%c \n",pg[i].proc);
pt1=pg[i].pt;
n=pg[i].n;
for(int k=0;k<n;++k)
{
printf("page no %d ",pgt[pt1].p[k]);
printf("frame no %d\n",pgt[pt1].f[k]);
}
}
}
void delproc()
{ char ch,pt,n,f;
printf("which process do u want to delete from memory\n");
scanf(" %c",&ch);
for(int i=0;i<7;++i)
if(pg[i].proc==ch)
{
pt=pg[i].pt;
n=pg[i].n;
for(int j=0;j<n;++j)
{
f=pgt[pt].f[j];
mem[f]=0;
pgt[pt].f[j]=0;
}
}
}
void pageload()
{
int j=0,n,temp;
printf("enetr the process name\n");
scanf(" %c",&pg[z].proc);
pg[z].pt=z;
printf("enetr the number of pages\n");
scanf("%d",&n);
pg[z].n=n;
printf("enetr page numbers\n");
for(int i=0;i<n;++i)
{
scanf("%d",&temp);
while(mem[j]!=0)
++j;
mem[j]=temp;
pgt[pg[z].pt].p[i]=temp;
pgt[pg[z].pt].f[i]=j;
}
++z;
}
|
CODING BY ~
Mr Ayub Khan
|